Welcome to the third part of our guide: "how to add extensions to iOS apps", where we’ll complete the table implementation in the main view of our iOS application.
In the previous part of our iPhone app development guide I explained how to:
- enable resource sharing in an app
- create a manager for saving and reading data
Table implementation
Let’s go back to our main ViewController
and finish implementing the table.
We already have a place to save our data and thanks to the manager we’ve created, data can now be added and removed within the view.
First off, implement these two protocols that will allow us to use our table:
UITableViewDelegate
and UITableViewDataSource
.
Our class should look like this:
import UIKit
class ViewController: UIViewController, UITableViewDelegate, UITableViewDataSource {
@IBOutlet weak var tableView: UITableView!
override func viewDidLoad() {
super.viewDidLoad()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
}
We should see an error message, what means that we have to add the missing delegates numberOfRowslnSection
and callForRowAtlndexPath
. Let’s do this!
First, add the delegates service and data source to our table. In viewDidLoad()
insert the following lines:
self.tableView.delegate = self
self.tableView.dataSource = self
Next, implement the required delegates.
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return LocalStorageManager.sharedInstance.readData().count
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = UITableViewCell()
cell.textLabel!.text = LocalStorageManager.sharedInstance.readData()[indexPath.row]
return cell
}
In the first one, determine the amount of rows per section (in this case we have only one section). In the next one, define the behavior of the cell row. The only thing left is to set the text using the indexPath.row
for returned table from our manager’s readData()
method.
If we would run our app now, we would only see lines separating particular table rows. Add manually a few entries just to see if everything works as expected.
In the viewDidLoad()
method, add three lines which will insert data to our container using our manager.
override func viewDidLoad() {
super.viewDidLoad()
LocalStorageManager.sharedInstance.insertData("Entry 1")
LocalStorageManager.sharedInstance.insertData("Entry 1")
LocalStorageManager.sharedInstance.insertData("Entry 1")
self.tableView.delegate = self
self.tableview.dataSource = self
}
If we run the app now, we’ll see three manually added positions in our table.
As you can see, our entries were added to the table so everything should work properly. Let’s replace these three lines in viewDidLoad()
with one that will clear all saved data.
override func viewDidLoad() {
super.viewDidLoad()
LocalStorageManager.sharedInstance.clearData()
self.tableView.delegate = self
self.tableview.dataSource = self
}
After running the app, our table should be empty. By the way, we should add the possibility to delete entries in the table through cell swiping.
Implement two required delegates:
func tableView(tableView: UITableView, editActionsForRowAtIndexPath indexPath: NSIndexPath) -> [UITableViewRowAction]? {
let remove = UITableViewRowAction(style: .Destructive, title: "Remove") { action, index in
LocalStorageManager.sharedInstance.removeData(index.row)
self.tableView.reloadData()
}
return [remove]
}
func tableView(tableView: UITableView, canEditRowAtIndexPath indexPath: NSIndexPath) -> Bool {
return true
}
In the first one, add a RowAction
with the title Remove
.
Then delete entries from our database. Remember to refresh the table with reloadData()
after deleting a line. In the second delegate, define which row should have the option of choosing an action, return “true” for all of them. Now, after running the iOS app, it will be possible to delete entries by swiping a line.
Next on “How to add extensions to iOS apps”:
In the last part of our iPhone app development guide we’ll cover adding and saving files.
You can have a look at the finished app in our GitHub repo here.
Popular posts

Offline Access in a Mobile App Used on Construction Sites
For the last few years, we have been working on a user-friendly construction project management app. This kind of tool includes an array of features, for example issuing tasks and document management. However, there is one crucial feature that is often overlooked but plays a vital role on construction sites: offline functionality.
Read more
Enhancing API Testing Efficiency with JavaScript in Postman
API testing is essential for good software, and Postman has made it easier by letting us use JavaScript to check our work. In this article, we'll see how using JavaScript in Postman helps us make sure our software is working right, saving us time and making our testing process smoother.
Read more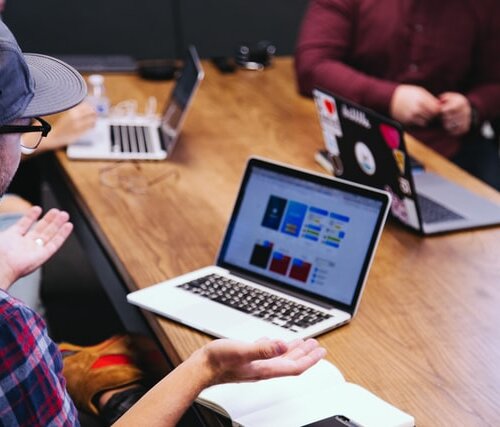
Flutter application template with GitHub Actions
This article explains how to create Flutter application template with GitHub Actions to save yourself time and nerves.
Read more