Learn how to add extensions to iOS apps to provide additional actions to your users without launching the app itself. In this part you’ll find out how to enable iOS app resource sharing and create a simple manager for saving and reading files.
In part one of our iPhone app development guide I wrote about:
- creating the app project
- programming the main view of iOS app
- adding an extension to iOS app
Shared resources
To enable resource sharing, set a common data container that can be used by your targets (the main app and the extension).
Beside the marked target, choose the Capabilities
bookmark. Select App Groups
form the list and run it. Click the “+” button and write the container name.
After approving you should see the new entry.
For now, we’ve just created a container for our iOS app targets. This whole process must be repeated for the main target of the app. So, on the left hand side, select AppExtensionSample
from the list of targets and repeat these actions (write the same name when adding).
Local Storage Manager
Now that we have a dedicated container to save our data from both app targets, let’s move on to creating a simple manager for saving and reading data.
In the main app target, create new class called LocalStorageManager
with the following code:
import UIKit
public class LocalStorageManager{
static let sharedInstance = LocalStorageManager()
private let sharedContainer: NSUserDefaults = NSUserDefaults(suiteName: "group.co.zaven.AppExtensionSample")!
private var storedData: [String]! = []
private init(){
self.readData()
}
func readData() -> [String]{
if let data = self.sharedContainer.objectForKey("FileList") as? [String] {
self.storedData = data
}
return self.storedData
}
func insertData(fileName: String){
self.storedData.append(fileName)
self.sharedContainer.setObject(self.storedData, forKey: "FileList")
}
func removeData(index: Int){
self.storedData.removeAtIndex(index)
self.sharedContainer.setObject(self.storedData, forKey: "FileList")
}
func clearData(){
self.storedData.removeAll()
self.sharedContainer.removeObjectForKey("FileList")
}
}
A simple singleton for using our local store. Pay attention to the sharedContainer
declaration, where I refer to the name of the already created group container. By default, we would refer to the standardUserDefaults
, that is allocated only to the one target in which we used it. This is how we’ll refer to a common container. Without firther obstacles, we’ll be able to save or read separated targets data.
Next on “How to add extensions to iOS apps”:
In the 3rd part of our iPhone app development guide, we will perform some final touches on the table implementation in the main application.
YOU CAN HAVE A LOOK AT THE FINISHED APP IN OUR GITHUB REPO HERE.
Popular posts

Enhancing API Testing Efficiency with JavaScript in Postman
API testing is essential for good software, and Postman has made it easier by letting us use JavaScript to check our work. In this article, we'll see how using JavaScript in Postman helps us make sure our software is working right, saving us time and making our testing process smoother.
Read more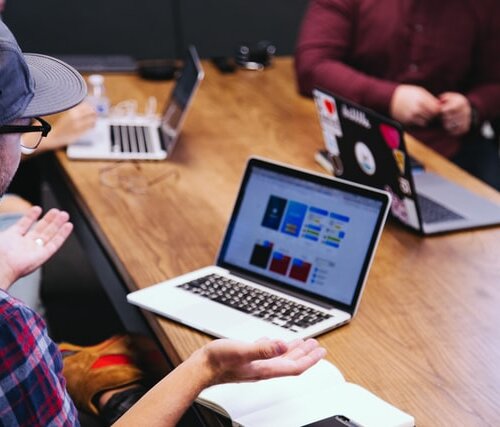
Flutter application template with GitHub Actions
This article explains how to create Flutter application template with GitHub Actions to save yourself time and nerves.
Read more
Augmented Reality as a trend in Mobile Development
3D maps, realistic games, virtual showrooms and fitting rooms, interactive participation in a conference or concert, visiting museums and exhibitions. These are just a few examples of where Augmented Reality can be used. Applications created with the help of AR effectively combine the real world with the virtual one. Can your business also benefit from […]
Read more